Logistic Regression Classifier - Fitting hospital-specific models
Contents
Logistic Regression Classifier - Fitting hospital-specific models#
Aims#
Assess accuracy of a logistic regression classifier, using k-fold (5-fold) training/test data splits (each data point is present in one and only one of the five test sets). This notebook fits models to each hospital independently.
The notebook includes:
A range of accuracy scores
Receiver operating characteristic (ROC) and Sensitivity-Specificity Curves
Import libraries#
# Turn warnings off to keep notebook tidy
import warnings
warnings.filterwarnings("ignore")
import matplotlib.pyplot as plt
from matplotlib.lines import Line2D
import numpy as np
import pandas as pd
from sklearn.preprocessing import StandardScaler
from sklearn.linear_model import LogisticRegression
from sklearn.metrics import auc
from sklearn.metrics import roc_curve
Load train and test set data#
K-fold train/test split has previously been performed. Train/test data is stratified by hospital and thrombolysis.
# Lists to hold data splits
train_splits = []
train_splits_one_hot_hosp = []
test_splits = []
test_splits_one_hot_hosp = []
# Loop to load data and add to lists
for i in range (5):
train_splits.append(pd.read_csv(f'../data/kfold_5fold/train_{i}.csv'))
test_splits.append(pd.read_csv(f'../data/kfold_5fold/test_{i}.csv'))
Functions#
Standardise data#
Standardisation subtracts the mean and divides by the standard deviation, for each feature. Here we use the sklearn built-in method for standardisation.
def standardise_data(X_train, X_test):
"""
Converts all data to a similar scale.
Standardisation subtracts mean and divides by standard deviation
for each feature.
Standardised data will have a mena of 0 and standard deviation of 1.
The training data mean and standard deviation is used to standardise both
training and test set data.
"""
# Initialise a new scaling object for normalising input data
sc = StandardScaler()
# Set up the scaler just on the training set
sc.fit(X_train)
# Apply the scaler to the training and test sets
train_std=sc.transform(X_train)
test_std=sc.transform(X_test)
return train_std, test_std
Find model probability threshold to match predicted and actual thrombolysis use#
def find_threshold(probabilities, true_rate):
"""
Find classification threshold to calibrate model
"""
index = (1-true_rate)*len(probabilities)
threshold = sorted(probabilities)[int(index)]
return threshold
Calculate accuracy measures#
def calculate_accuracy(observed, predicted):
"""
Calculates a range of accuracy scores from observed and predicted classes.
Takes two list or NumPy arrays (observed class values, and predicted class
values), and returns a dictionary of results.
1) observed positive rate: proportion of observed cases that are +ve
2) Predicted positive rate: proportion of predicted cases that are +ve
3) observed negative rate: proportion of observed cases that are -ve
4) Predicted negative rate: proportion of predicted cases that are -ve
5) accuracy: proportion of predicted results that are correct
6) precision: proportion of predicted +ve that are correct
7) recall: proportion of true +ve correctly identified
8) f1: harmonic mean of precision and recall
9) sensitivity: Same as recall
10) specificity: Proportion of true -ve identified:
11) positive likelihood: increased probability of true +ve if test +ve
12) negative likelihood: reduced probability of true +ve if test -ve
13) false positive rate: proportion of false +ves in true -ve patients
14) false negative rate: proportion of false -ves in true +ve patients
15) true positive rate: Same as recall
16) true negative rate: Same as specificity
17) positive predictive value: chance of true +ve if test +ve
18) negative predictive value: chance of true -ve if test -ve
"""
# Converts list to NumPy arrays
if type(observed) == list:
observed = np.array(observed)
if type(predicted) == list:
predicted = np.array(predicted)
# Calculate accuracy scores
observed_positives = observed == 1
observed_negatives = observed == 0
predicted_positives = predicted == 1
predicted_negatives = predicted == 0
true_positives = (predicted_positives == 1) & (observed_positives == 1)
false_positives = (predicted_positives == 1) & (observed_positives == 0)
true_negatives = (predicted_negatives == 1) & (observed_negatives == 1)
false_negatives = (predicted_negatives == 1) & (observed_negatives == 0)
accuracy = np.mean(predicted == observed)
precision = (np.sum(true_positives) /
(np.sum(true_positives) + np.sum(false_positives)))
recall = np.sum(true_positives) / np.sum(observed_positives)
sensitivity = recall
f1 = 2 * ((precision * recall) / (precision + recall))
specificity = np.sum(true_negatives) / np.sum(observed_negatives)
positive_likelihood = sensitivity / (1 - specificity)
negative_likelihood = (1 - sensitivity) / specificity
false_positive_rate = 1 - specificity
false_negative_rate = 1 - sensitivity
true_positive_rate = sensitivity
true_negative_rate = specificity
positive_predictive_value = (np.sum(true_positives) /
(np.sum(true_positives) + np.sum(false_positives)))
negative_predictive_value = (np.sum(true_negatives) /
(np.sum(true_negatives) + np.sum(false_negatives)))
# Create dictionary for results, and add results
results = dict()
results['observed_positive_rate'] = np.mean(observed_positives)
results['observed_negative_rate'] = np.mean(observed_negatives)
results['predicted_positive_rate'] = np.mean(predicted_positives)
results['predicted_negative_rate'] = np.mean(predicted_negatives)
results['accuracy'] = accuracy
results['precision'] = precision
results['recall'] = recall
results['f1'] = f1
results['sensitivity'] = sensitivity
results['specificity'] = specificity
results['positive_likelihood'] = positive_likelihood
results['negative_likelihood'] = negative_likelihood
results['false_positive_rate'] = false_positive_rate
results['false_negative_rate'] = false_negative_rate
results['true_positive_rate'] = true_positive_rate
results['true_negative_rate'] = true_negative_rate
results['positive_predictive_value'] = positive_predictive_value
results['negative_predictive_value'] = negative_predictive_value
return results
Line intersect#
Used to find point of sensitivity-specificity curve where sensitivity = specificity.
def get_intersect(a1, a2, b1, b2):
"""
Returns the point of intersection of the lines passing through a2,a1 and b2,b1.
a1: [x, y] a point on the first line
a2: [x, y] another point on the first line
b1: [x, y] a point on the second line
b2: [x, y] another point on the second line
"""
s = np.vstack([a1,a2,b1,b2]) # s for stacked
h = np.hstack((s, np.ones((4, 1)))) # h for homogeneous
l1 = np.cross(h[0], h[1]) # get first line
l2 = np.cross(h[2], h[3]) # get second line
x, y, z = np.cross(l1, l2) # point of intersection
if z == 0: # lines are parallel
return (float('inf'), float('inf'))
return (x/z, y/z)
Fit hospital-specific models#
hospitals = set(train_splits[0]['StrokeTeam'])
# Set up list to store models and calibarion thresholds
hospital_models = []
thresholds = []
# Set up lists for results
observed = []
predicted_proba = []
predicted = []
kfold_result = []
hospital_results = []
threshold_results = []
feature_data = []
# Loop through k folds
for k_fold in range(5):
# Get k fold split
train = train_splits[k_fold]
test = test_splits[k_fold]
# Loop through hospitals
for hospital in hospitals:
# Get X and y
mask = train['StrokeTeam'] == hospital
X_train = train[mask].drop(['S2Thrombolysis', 'StrokeTeam'], axis=1)
y_train = train.loc[mask]['S2Thrombolysis']
mask = test['StrokeTeam'] == hospital
X_test = test[mask].drop(['S2Thrombolysis', 'StrokeTeam'], axis=1)
y_test = test.loc[mask]['S2Thrombolysis']
feature_data.append(test[mask])
# Standardise X data
X_train_std, X_test_std = standardise_data(X_train, X_test)
# Define and Fit model
model = LogisticRegression(solver='lbfgs')
model.fit(X_train_std, y_train)
# Get predicted probabilities
y_probs = model.predict_proba(X_test_std)[:,1]
# Calibrate model and get class
true_rate = np.mean(y_test)
threshold = find_threshold(y_probs, true_rate)
thresholds.append(threshold)
y_class = y_probs >= threshold
y_class = np.array(y_class) * 1.0
# Store results
observed.extend(list(y_test))
predicted_proba.extend(list(y_probs))
predicted.extend(y_class)
kfold_result.extend(list(np.repeat(k_fold, len(y_test))))
hospital_results.extend(list(np.repeat(hospital, len(y_test))))
threshold_results.extend(np.repeat(threshold, len(y_test)))
# Collate patient-level results
multi_model = pd.DataFrame()
multi_model['hospital'] = hospital_results
multi_model['observed'] = np.array(observed) * 1.0
multi_model['prob'] = predicted_proba
multi_model['predicted'] = predicted
multi_model['k_fold'] = kfold_result
multi_model['threshold'] = threshold_results
multi_model['correct'] = multi_model['observed'] == multi_model['predicted']
# Save model
filename = './predictions/multi_fit_lr_k_fold.csv'
multi_model.to_csv(filename, index=False)
# Save features
feature_data_df = pd.concat(feature_data, axis=0)
feature_data_df.to_csv('./predictions/feature_data.csv')
Results#
Accuracy measures#
k_fold_results = []
for i in range(5):
mask = multi_model['k_fold'] == i
mask = mask.values
observed = multi_model.loc[mask]['observed']
predicted = multi_model.loc[mask]['predicted']
results = calculate_accuracy(observed, predicted)
k_fold_results.append(results)
multi_fit_results = pd.DataFrame(k_fold_results).T
multi_fit_results
0 | 1 | 2 | 3 | 4 | |
---|---|---|---|---|---|
observed_positive_rate | 0.295232 | 0.295401 | 0.295176 | 0.295080 | 0.295417 |
observed_negative_rate | 0.704768 | 0.704599 | 0.704824 | 0.704920 | 0.704583 |
predicted_positive_rate | 0.295570 | 0.295907 | 0.295907 | 0.295699 | 0.295980 |
predicted_negative_rate | 0.704430 | 0.704093 | 0.704093 | 0.704301 | 0.704020 |
accuracy | 0.807489 | 0.805971 | 0.802373 | 0.807197 | 0.805342 |
precision | 0.673768 | 0.671290 | 0.664830 | 0.672942 | 0.670213 |
recall | 0.674538 | 0.672440 | 0.666476 | 0.674352 | 0.671488 |
f1 | 0.674153 | 0.671865 | 0.665652 | 0.673646 | 0.670850 |
sensitivity | 0.674538 | 0.672440 | 0.666476 | 0.674352 | 0.671488 |
specificity | 0.863183 | 0.861953 | 0.859285 | 0.862806 | 0.861464 |
positive_likelihood | 4.930225 | 4.871109 | 4.736364 | 4.915321 | 4.847017 |
negative_likelihood | 0.377048 | 0.380020 | 0.388141 | 0.377429 | 0.381341 |
false_positive_rate | 0.136817 | 0.138047 | 0.140715 | 0.137194 | 0.138536 |
false_negative_rate | 0.325462 | 0.327560 | 0.333524 | 0.325648 | 0.328512 |
true_positive_rate | 0.674538 | 0.672440 | 0.666476 | 0.674352 | 0.671488 |
true_negative_rate | 0.863183 | 0.861953 | 0.859285 | 0.862806 | 0.861464 |
positive_predictive_value | 0.673768 | 0.671290 | 0.664830 | 0.672942 | 0.670213 |
negative_predictive_value | 0.863596 | 0.862573 | 0.860177 | 0.863564 | 0.862152 |
multi_fit_results.T.describe()
observed_positive_rate | observed_negative_rate | predicted_positive_rate | predicted_negative_rate | accuracy | precision | recall | f1 | sensitivity | specificity | positive_likelihood | negative_likelihood | false_positive_rate | false_negative_rate | true_positive_rate | true_negative_rate | positive_predictive_value | negative_predictive_value | |
---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|
count | 5.000000 | 5.000000 | 5.000000 | 5.000000 | 5.000000 | 5.000000 | 5.000000 | 5.000000 | 5.000000 | 5.000000 | 5.000000 | 5.000000 | 5.000000 | 5.000000 | 5.000000 | 5.000000 | 5.000000 | 5.000000 |
mean | 0.295261 | 0.704739 | 0.295812 | 0.704188 | 0.805674 | 0.670609 | 0.671859 | 0.671233 | 0.671859 | 0.861738 | 4.860007 | 0.380796 | 0.138262 | 0.328141 | 0.671859 | 0.861738 | 0.670609 | 0.862412 |
std | 0.000146 | 0.000146 | 0.000172 | 0.000172 | 0.002044 | 0.003516 | 0.003273 | 0.003393 | 0.003273 | 0.001530 | 0.076763 | 0.004479 | 0.001530 | 0.003273 | 0.003273 | 0.001530 | 0.003516 | 0.001398 |
min | 0.295080 | 0.704583 | 0.295570 | 0.704020 | 0.802373 | 0.664830 | 0.666476 | 0.665652 | 0.666476 | 0.859285 | 4.736364 | 0.377048 | 0.136817 | 0.325462 | 0.666476 | 0.859285 | 0.664830 | 0.860177 |
25% | 0.295176 | 0.704599 | 0.295699 | 0.704093 | 0.805342 | 0.670213 | 0.671488 | 0.670850 | 0.671488 | 0.861464 | 4.847017 | 0.377429 | 0.137194 | 0.325648 | 0.671488 | 0.861464 | 0.670213 | 0.862152 |
50% | 0.295232 | 0.704768 | 0.295907 | 0.704093 | 0.805971 | 0.671290 | 0.672440 | 0.671865 | 0.672440 | 0.861953 | 4.871109 | 0.380020 | 0.138047 | 0.327560 | 0.672440 | 0.861953 | 0.671290 | 0.862573 |
75% | 0.295401 | 0.704824 | 0.295907 | 0.704301 | 0.807197 | 0.672942 | 0.674352 | 0.673646 | 0.674352 | 0.862806 | 4.915321 | 0.381341 | 0.138536 | 0.328512 | 0.674352 | 0.862806 | 0.672942 | 0.863564 |
max | 0.295417 | 0.704920 | 0.295980 | 0.704430 | 0.807489 | 0.673768 | 0.674538 | 0.674153 | 0.674538 | 0.863183 | 4.930225 | 0.388141 | 0.140715 | 0.333524 | 0.674538 | 0.863183 | 0.673768 | 0.863596 |
Receiver Operator Characteristic and Sensitivity-Specificity Curves#
Receiver Operator Characteristic Curve:
k_fold_fpr = []
k_fold_tpr = []
k_fold_thresholds = []
k_fold_auc = []
for i in range(5):
mask = multi_model['k_fold'] == i
mask = mask.values
observed = multi_model.loc[mask]['observed']
predicted_proba = multi_model.loc[mask]['prob']
fpr, tpr, thresholds = roc_curve(observed, predicted_proba)
roc_auc = auc(fpr, tpr)
k_fold_fpr.append(fpr)
k_fold_tpr.append(tpr)
k_fold_thresholds.append(thresholds)
k_fold_auc.append(roc_auc)
print (f'Run {i} AUC {roc_auc:0.4f}')
# Show mean area under curve
mean_auc = np.mean(k_fold_auc)
sd_auc = np.std(k_fold_auc)
print (f'\nMean AUC: {mean_auc:0.4f}')
print (f'SD AUC: {sd_auc:0.4f}')
Run 0 AUC 0.8710
Run 1 AUC 0.8695
Run 2 AUC 0.8688
Run 3 AUC 0.8705
Run 4 AUC 0.8715
Mean AUC: 0.8702
SD AUC: 0.0010
Sensitivity-specificity curve:
k_fold_sensitivity = []
k_fold_specificity = []
for i in range(5):
# Get classificiation probabilities for k-fold replicate
mask = multi_model['k_fold'] == i
mask = mask.values
observed = multi_model.loc[mask]['observed']
predicted_proba = multi_model.loc[mask]['prob']
# Set up list for accuracy measures
sensitivity = []
specificity = []
# Loop through increments in probability of survival
thresholds = np.arange(0.0, 1.01, 0.01)
for cutoff in thresholds: # loop 0 --> 1 on steps of 0.1
# Get classificiation using cutoff
predicted_class = predicted_proba >= cutoff
predicted_class = predicted_class.values * 1.0
# Call accuracy measures function
accuracy = calculate_accuracy(observed, predicted_class)
# Add accuracy scores to lists
sensitivity.append(accuracy['sensitivity'])
specificity.append(accuracy['specificity'])
# Add replicate to lists
k_fold_sensitivity.append(sensitivity)
k_fold_specificity.append(specificity)
Combined plot:
fig = plt.figure(figsize=(10,5))
# Plot ROC
ax1 = fig.add_subplot(121)
for i in range(5):
ax1.plot(k_fold_fpr[i], k_fold_tpr[i], color='orange')
ax1.plot([0, 1], [0, 1], color='darkblue', linestyle='--')
ax1.set_xlabel('False Positive Rate')
ax1.set_ylabel('True Positive Rate')
ax1.set_title('Receiver Operator Characteristic Curve')
text = f'Mean AUC: {mean_auc:.3f}'
ax1.text(0.64,0.07, text,
bbox=dict(facecolor='white', edgecolor='black'))
plt.grid(True)
# Plot sensitivity-specificity
ax2 = fig.add_subplot(122)
for i in range(5):
ax2.plot(k_fold_sensitivity[i], k_fold_specificity[i])
ax2.set_xlabel('Sensitivity')
ax2.set_ylabel('Specificity')
ax2.set_title('Sensitivity-Specificity Curve')
plt.grid(True)
plt.tight_layout(pad=2)
plt.savefig('./output/lr_hospital_fit_roc_sens_spec.jpg', dpi=300)
plt.show()
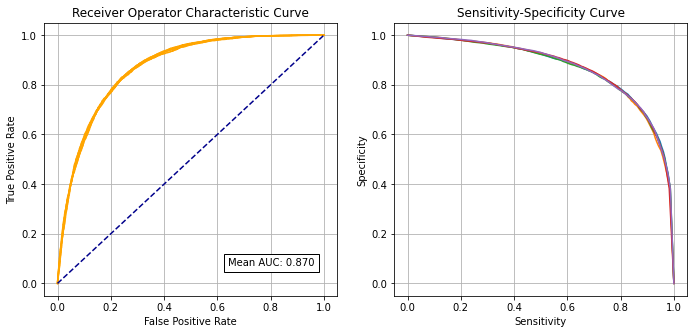
Identify cross-over of sensitivity and specificity#
sens = np.array(k_fold_sensitivity).mean(axis=0)
spec = np.array(k_fold_specificity).mean(axis=0)
df = pd.DataFrame()
df['sensitivity'] = sens
df['specificity'] = spec
df['spec greater sens'] = spec > sens
# find last index for senitivity being greater than specificity
mask = df['spec greater sens'] == False
last_id_sens_greater_spec = np.max(df[mask].index)
locs = [last_id_sens_greater_spec, last_id_sens_greater_spec + 1]
points = df.iloc[locs][['sensitivity', 'specificity']]
# Get intersetction with line of x=y
a1 = list(points.iloc[0].values)
a2 = list(points.iloc[1].values)
b1 = [0, 0]
b2 = [1, 1]
intersect = get_intersect(a1, a2, b1, b2)[0]
print(f'\nIntersect: {intersect:0.3f}')
Intersect: 0.789
Calibration and assessment of accuracy when model has high confidence#
# Collate results in Dataframe
reliability_collated = pd.DataFrame()
# Loop through k fold predictions
for i in range(5):
# Get observed class and predicted probability
mask = multi_model['k_fold'] == i
obs = multi_model[mask]['observed'].values
prob = multi_model[mask]['prob'].values
# Bin data with numpy digitize (this will assign a bin to each case)
step = 0.10
bins = np.arange(step, 1+step, step)
digitized = np.digitize(prob, bins)
# Put single fold data in DataFrame
reliability = pd.DataFrame()
reliability['bin'] = digitized
reliability['probability'] = prob
reliability['observed'] = obs
classification = 1 * (prob > 0.5 )
reliability['correct'] = obs == classification
reliability['count'] = 1
# Summarise data by bin in new dataframe
reliability_summary = pd.DataFrame()
# Add bins and k-fold to summary
reliability_summary['bin'] = bins
reliability_summary['k-fold'] = i
# Calculate mean of predicted probability of thrombolysis in each bin
reliability_summary['confidence'] = \
reliability.groupby('bin').mean()['probability']
# Calculate the proportion of patients who receive thrombolysis
reliability_summary['fraction_positive'] = \
reliability.groupby('bin').mean()['observed']
# Calculate proportion correct in each bin
reliability_summary['fraction_correct'] = reliability.groupby('bin').mean()['correct']
# Calculate fraction of results in each bin
reliability_summary['fraction_results'] = \
reliability.groupby('bin').sum()['count'] / reliability.shape[0]
# Add k-fold results to DatafRame collation
reliability_collated = reliability_collated.append(reliability_summary)
# Get mean results
reliability_summary = reliability_collated.groupby('bin').mean()
reliability_summary.drop('k-fold', axis=1, inplace=True)
reliability_summary
confidence | fraction_positive | fraction_correct | fraction_results | |
---|---|---|---|---|
bin | ||||
0.1 | 0.018772 | 0.049217 | 0.950783 | 0.458798 |
0.2 | 0.146496 | 0.206722 | 0.793278 | 0.091344 |
0.3 | 0.248232 | 0.304989 | 0.695011 | 0.065862 |
0.4 | 0.349055 | 0.369431 | 0.630569 | 0.055506 |
0.5 | 0.448903 | 0.456114 | 0.543886 | 0.051187 |
0.6 | 0.549545 | 0.525635 | 0.525635 | 0.047836 |
0.7 | 0.650405 | 0.586572 | 0.586572 | 0.050445 |
0.8 | 0.749642 | 0.674949 | 0.674949 | 0.047724 |
0.9 | 0.851498 | 0.746320 | 0.746320 | 0.056596 |
1.0 | 0.953730 | 0.812274 | 0.812274 | 0.074701 |
fig = plt.figure(figsize=(10,5))
# Plot predicted prob vs fraction psotive
ax1 = fig.add_subplot(1,2,1)
# Loop through k-fold reliability results
for i in range(5):
mask = reliability_collated['k-fold'] == i
k_fold_result = reliability_collated[mask]
x = k_fold_result['confidence']
y = k_fold_result['fraction_positive']
ax1.plot(x,y, color='orange')
# Add 1:1 line
ax1.plot([0,1],[0,1], color='k', linestyle ='--')
# Refine plot
ax1.set_xlabel('Model probability')
ax1.set_ylabel('Fraction positive')
ax1.set_xlim(0, 1)
ax1.set_ylim(0, 1)
ax1.grid()
# Plot accuracy vs probability
ax2 = fig.add_subplot(1,2,2)
# Loop through k-fold reliability results
for i in range(5):
mask = reliability_collated['k-fold'] == i
k_fold_result = reliability_collated[mask]
x = k_fold_result['confidence']
y = k_fold_result['fraction_correct']
ax2.plot(x,y, color='orange')
# Refine plot
ax2.set_xlabel('Model probability')
ax2.set_ylabel('Fraction correct')
ax2.set_xlim(0, 1)
ax2.set_ylim(0, 1)
ax2.grid()
ax3 = ax2.twinx() # instantiate a second axes that shares the same x-axis
for i in range(5):
mask = reliability_collated['k-fold'] == i
k_fold_result = reliability_collated[mask]
x = k_fold_result['confidence']
y = k_fold_result['fraction_results']
ax3.plot(x,y, color='blue')
ax3.set_xlim(0, 1)
ax3.set_ylim(0, 0.5)
ax3.set_ylabel('Fraction of samples')
custom_lines = [Line2D([0], [0], color='orange', alpha=0.6, lw=2),
Line2D([0], [0], color='blue', alpha = 0.6,lw=2)]
plt.legend(custom_lines, ['Fraction correct', 'Fraction of samples'],
loc='upper center')
plt.tight_layout(pad=2)
plt.savefig('./output/lr_hospital_fit_reliability.jpg', dpi=300)
plt.show()
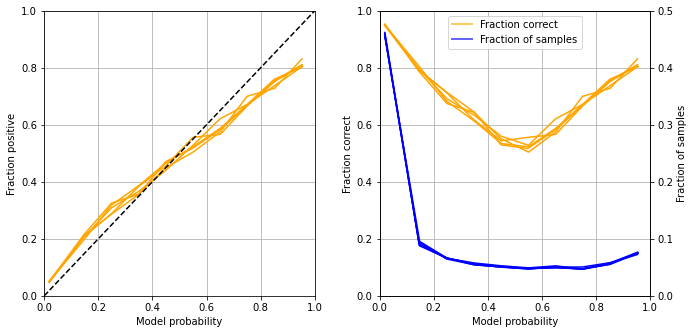
Get accuracy of model when model is at least 80% confident
bins = [0.1, 0.2, 0.9, 1.0]
acc = reliability_summary.loc[bins].mean()['fraction_correct']
frac = reliability_summary.loc[bins].sum()['fraction_results']
print ('For For example,samples with at least 80% confidence:')
print (f'Proportion of all samples: {frac:0.3f}')
print (f'Accuracy: {acc:0.3f}')
For For example,samples with at least 80% confidence:
Proportion of all samples: 0.681
Accuracy: 0.826
Observations#
Fitting at a single hospital level gave an accuracy of 80.7% (c.f. 83.2% for a single model), and a ROC AUC of 0.870 (c.f. 0.904 for a single fit model)
Accuracy was 82.6% for the 68% of samples with at least 80% confidence of model
The model can achieve 78.9% sensitivity and specificity simultaneously
ROC ACUC = 0.870
The model showed poorer calibration than a single model