Modular TensorFlow model with 2D embedding - analyse
Contents
Modular TensorFlow model with 2D embedding - analyse#
Embedding converts a categorical variable into a projection onto n-dimensional space [1], and has been shown to be an effective way to train neural network when using categorical data, while also allowing a measure of similarity/distance between different values of the categorical data, Here we use embedding for hospital ID. We also convert patient data and pathway data into an embedded vector (this may also be known as encoding the data in a vector with fewer dimensions than the original data set for those groups of features).
[1] Guo C, & Berkhahn F. (2016) Entity Embeddings of Categorical Variables. arXiv:160406737 [cs] http://arxiv.org/abs/1604.06737
Pre-trained models from 002b_1d_modular_fit.ipynb
Models are fitted to previously split training and test data sets.
Aims#
Test performance of model using subnets for:
Patient clinical data: Age, gender, ethnicity, disability before stroke, stroke scale data. Pass through one hidden layer (with 2x neurons as input features) and then to two neurons with sigmoid activation.
Pathway process data: Times of arrival and scan, time of day, day of week. Pass through one hidden layer (with 2x neurons as input features) and then to two neurons with sigmoid activation.
Hospital ID (one-hot encoded): Connect input directly to two neurons with sigmoid activation.
Import libraries#
path = './saved_models/2d_modular/'
# Turn warnings off to keep notebook tidy
import warnings
warnings.filterwarnings("ignore")
import matplotlib.pyplot as plt
from matplotlib.lines import Line2D
import numpy as np
import os
import pandas as pd
# sklearn for pre-processing
from sklearn.preprocessing import MinMaxScaler
from sklearn.metrics import auc
# TensorFlow api model
from tensorflow import keras
from tensorflow.keras import layers
from tensorflow.keras.models import Model
from tensorflow.keras.optimizers import Adam
from tensorflow.keras import backend as K
from tensorflow.keras.losses import binary_crossentropy
Import data#
train_data, test_data = [], []
data_loc = '../data/kfold_5fold/'
for i in range(5):
train_data.append(pd.read_csv(data_loc + 'train_{0}.csv'.format(i)))
test_data.append(pd.read_csv(data_loc + 'test_{0}.csv'.format(i)))
Define function to scale data#
def scale_data(X_train, X_test):
"""Scale data 0-1 based on min and max in training set"""
# Initialise a new scaling object for normalising input data
sc = MinMaxScaler()
# Set up the scaler just on the training set
sc.fit(X_train)
# Apply the scaler to the training and test sets
train_sc = sc.transform(X_train)
test_sc = sc.transform(X_test)
return train_sc, test_sc
Define function for accuracy#
def calculate_accuracy(observed, predicted):
"""
Calculates a range of accuracy scores from observed and predicted classes.
Takes two list or NumPy arrays (observed class values, and predicted class
values), and returns a dictionary of results.
1) observed positive rate: proportion of observed cases that are +ve
2) Predicted positive rate: proportion of predicted cases that are +ve
3) observed negative rate: proportion of observed cases that are -ve
4) Predicted negative rate: proportion of predicted cases that are -ve
5) accuracy: proportion of predicted results that are correct
6) precision: proportion of predicted +ve that are correct
7) recall: proportion of true +ve correctly identified
8) f1: harmonic mean of precision and recall
9) sensitivity: Same as recall
10) specificity: Proportion of true -ve identified:
11) positive likelihood: increased probability of true +ve if test +ve
12) negative likelihood: reduced probability of true +ve if test -ve
13) false positive rate: proportion of false +ves in true -ve patients
14) false negative rate: proportion of false -ves in true +ve patients
15) true positive rate: Same as recall
16) true negative rate: Same as specificity
17) positive predictive value: chance of true +ve if test +ve
18) negative predictive value: chance of true -ve if test -ve
"""
# Converts list to NumPy arrays
if type(observed) == list:
observed = np.array(observed)
if type(predicted) == list:
predicted = np.array(predicted)
# Calculate accuracy scores
observed_positives = observed == 1
observed_negatives = observed == 0
predicted_positives = predicted == 1
predicted_negatives = predicted == 0
true_positives = (predicted_positives == 1) & (observed_positives == 1)
false_positives = (predicted_positives == 1) & (observed_positives == 0)
true_negatives = (predicted_negatives == 1) & (observed_negatives == 1)
false_negatives = (predicted_negatives == 1) & (observed_negatives == 0)
accuracy = np.mean(predicted == observed)
precision = (np.sum(true_positives) /
(np.sum(true_positives) + np.sum(false_positives)))
recall = np.sum(true_positives) / np.sum(observed_positives)
sensitivity = recall
f1 = 2 * ((precision * recall) / (precision + recall))
specificity = np.sum(true_negatives) / np.sum(observed_negatives)
positive_likelihood = sensitivity / (1 - specificity)
negative_likelihood = (1 - sensitivity) / specificity
false_positive_rate = 1 - specificity
false_negative_rate = 1 - sensitivity
true_positive_rate = sensitivity
true_negative_rate = specificity
positive_predictive_value = (np.sum(true_positives) /
(np.sum(true_positives) + np.sum(false_positives)))
negative_predictive_value = (np.sum(true_negatives) /
(np.sum(true_negatives) + np.sum(false_negatives)))
# Create dictionary for results, and add results
results = dict()
results['observed_positive_rate'] = np.mean(observed_positives)
results['observed_negative_rate'] = np.mean(observed_negatives)
results['predicted_positive_rate'] = np.mean(predicted_positives)
results['predicted_negative_rate'] = np.mean(predicted_negatives)
results['accuracy'] = accuracy
results['precision'] = precision
results['recall'] = recall
results['f1'] = f1
results['sensitivity'] = sensitivity
results['specificity'] = specificity
results['positive_likelihood'] = positive_likelihood
results['negative_likelihood'] = negative_likelihood
results['false_positive_rate'] = false_positive_rate
results['false_negative_rate'] = false_negative_rate
results['true_positive_rate'] = true_positive_rate
results['true_negative_rate'] = true_negative_rate
results['positive_predictive_value'] = positive_predictive_value
results['negative_predictive_value'] = negative_predictive_value
return results
Define function for line intersect#
Used to find point of sensitivity-specificty curve where sensitivity = specificity.
def get_intersect(a1, a2, b1, b2):
"""
Returns the point of intersection of the lines passing through a2,a1 and b2,b1.
a1: [x, y] a point on the first line
a2: [x, y] another point on the first line
b1: [x, y] a point on the second line
b2: [x, y] another point on the second line
"""
s = np.vstack([a1,a2,b1,b2]) # s for stacked
h = np.hstack((s, np.ones((4, 1)))) # h for homogeneous
l1 = np.cross(h[0], h[1]) # get first line
l2 = np.cross(h[2], h[3]) # get second line
x, y, z = np.cross(l1, l2) # point of intersection
if z == 0: # lines are parallel
return (float('inf'), float('inf'))
return (x/z, y/z)
Use trained models to predict outcome of test data sets#
# Set up lists for accuracies and ROC data
accuracies = []
roc_fpr = []
roc_tpr = []
# Set up lists for observed and predicted
observed = []
predicted_proba = []
predicted = []
# Get data subgroups
subgroups = pd.read_csv('../data/subnet.csv', index_col='Item')
# Get list of clinical items
clinical_subgroup = subgroups.loc[subgroups['Subnet']=='clinical']
clinical_subgroup = list(clinical_subgroup.index)
# Get list of pathway items
pathway_subgroup = subgroups.loc[subgroups['Subnet']=='pathway']
pathway_subgroup = list(pathway_subgroup.index)
# Get list of hospital items
hospital_subgroup = subgroups.loc[subgroups['Subnet']=='hospital']
hospital_subgroup = list(hospital_subgroup.index)
# Loop through 5 k-folds
for k in range(5):
# Load data
train = pd.read_csv(f'../data/kfold_5fold/train_{k}.csv')
test = pd.read_csv(f'../data/kfold_5fold/test_{k}.csv')
# OneHot encode stroke team
coded = pd.get_dummies(train['StrokeTeam'])
train = pd.concat([train, coded], axis=1)
train.drop('StrokeTeam', inplace=True, axis=1)
coded = pd.get_dummies(test['StrokeTeam'])
test = pd.concat([test, coded], axis=1)
test.drop('StrokeTeam', inplace=True, axis=1)
# Split into X, y
X_train_df = train.drop('S2Thrombolysis',axis=1)
y_train_df = train['S2Thrombolysis']
X_test_df = test.drop('S2Thrombolysis',axis=1)
y_test_df = test['S2Thrombolysis']
# Split train and test data by subgroups
X_train_patients = X_train_df[clinical_subgroup]
X_test_patients = X_test_df[clinical_subgroup]
X_train_pathway = X_train_df[pathway_subgroup]
X_test_pathway = X_test_df[pathway_subgroup]
X_train_hospitals = X_train_df[hospital_subgroup]
X_test_hospitals = X_test_df[hospital_subgroup]
# Convert to NumPy
X_train = X_train_df.values
X_test = X_test_df.values
y_train = y_train_df.values
y_test = y_test_df.values
# Scale data
X_train_patients_sc, X_test_patients_sc = \
scale_data(X_train_patients, X_test_patients)
X_train_pathway_sc, X_test_pathway_sc = \
scale_data(X_train_pathway, X_test_pathway)
X_train_hospitals_sc, X_test_hospitals_sc = \
scale_data(X_train_hospitals, X_test_hospitals)
# Load model
filename = f'{path}model_{str(k)}.h5'
model = keras.models.load_model(filename)
# Get and store probablity
probability = model.predict(
[X_test_patients_sc, X_test_pathway_sc, X_test_hospitals_sc])
observed.append(y_test)
predicted_proba.append(probability.flatten())
# Get and store class
y_pred_class = probability >= 0.5
y_pred_class = y_pred_class.flatten()
predicted.append(y_pred_class)
# Get accuracy measurements
accuracy_dict = calculate_accuracy(y_test, y_pred_class)
accuracies.append(accuracy_dict)
# ROC
curve_fpr = [] # false positive rate
curve_tpr = [] # true positive rate
# Loop through increments in probability of survival
thresholds = np.arange(0, 1.01, 0.01)
for cutoff in thresholds: # loop 0 --> 1 on steps of 0.1
# Get whether passengers survive using cutoff
predicted_class = probability >= cutoff
predicted_class = predicted_class.flatten() * 1.0
# Call accuracy measures function
accuracy = calculate_accuracy(y_test, predicted_class)
# Add accuracy scores to lists
curve_fpr.append(accuracy['false_positive_rate'])
curve_tpr.append(accuracy['true_positive_rate'])
# Add roc to overall lists
roc_fpr.append(curve_fpr)
roc_tpr.append(curve_tpr)
results = pd.DataFrame(accuracies)
results.describe().T
count | mean | std | min | 25% | 50% | 75% | max | |
---|---|---|---|---|---|---|---|---|
observed_positive_rate | 5.0 | 0.295261 | 0.000146 | 0.295080 | 0.295176 | 0.295232 | 0.295401 | 0.295417 |
observed_negative_rate | 5.0 | 0.704739 | 0.000146 | 0.704583 | 0.704599 | 0.704768 | 0.704824 | 0.704920 |
predicted_positive_rate | 5.0 | 0.296003 | 0.006782 | 0.291313 | 0.291915 | 0.293827 | 0.295136 | 0.307826 |
predicted_negative_rate | 5.0 | 0.703997 | 0.006782 | 0.692174 | 0.704864 | 0.706173 | 0.708085 | 0.708687 |
accuracy | 5.0 | 0.851588 | 0.001871 | 0.849367 | 0.850613 | 0.851288 | 0.852356 | 0.854315 |
precision | 5.0 | 0.748160 | 0.006558 | 0.736804 | 0.748504 | 0.750963 | 0.751435 | 0.753096 |
recall | 5.0 | 0.749936 | 0.011751 | 0.738104 | 0.742525 | 0.747431 | 0.753239 | 0.768381 |
f1 | 5.0 | 0.748969 | 0.004069 | 0.743268 | 0.746720 | 0.749427 | 0.752261 | 0.753168 |
sensitivity | 5.0 | 0.749936 | 0.011751 | 0.738104 | 0.742525 | 0.747431 | 0.753239 | 0.768381 |
specificity | 5.0 | 0.894178 | 0.005111 | 0.885051 | 0.896018 | 0.896345 | 0.896626 | 0.896849 |
positive_likelihood | 5.0 | 7.095731 | 0.239413 | 6.684541 | 7.098377 | 7.198417 | 7.210777 | 7.286544 |
negative_likelihood | 5.0 | 0.279613 | 0.011847 | 0.261701 | 0.275210 | 0.281777 | 0.287088 | 0.292288 |
false_positive_rate | 5.0 | 0.105822 | 0.005111 | 0.103151 | 0.103374 | 0.103655 | 0.103982 | 0.114949 |
false_negative_rate | 5.0 | 0.250064 | 0.011751 | 0.231619 | 0.246761 | 0.252569 | 0.257475 | 0.261896 |
true_positive_rate | 5.0 | 0.749936 | 0.011751 | 0.738104 | 0.742525 | 0.747431 | 0.753239 | 0.768381 |
true_negative_rate | 5.0 | 0.894178 | 0.005111 | 0.885051 | 0.896018 | 0.896345 | 0.896626 | 0.896849 |
positive_predictive_value | 5.0 | 0.748160 | 0.006558 | 0.736804 | 0.748504 | 0.750963 | 0.751435 | 0.753096 |
negative_predictive_value | 5.0 | 0.895149 | 0.004028 | 0.890828 | 0.892647 | 0.894347 | 0.896698 | 0.901227 |
Receiver Operator Characteristic (ROC) Curve and Sensitivity-Specificity Curves#
Calculate areas of ROC curves.
k_fold_auc = []
for k in range(5):
# Get AUC
area = auc(roc_fpr[k], roc_tpr[k])
print (f'ROC AUC: {area:0.3f}')
k_fold_auc.append(area)
# Show mean area under curve
mean_auc = np.mean(k_fold_auc)
sd_auc = np.std(k_fold_auc)
print (f'\nMean AUC: {mean_auc:0.4f}')
print (f'SD AUC: {sd_auc:0.4f}')
ROC AUC: 0.920
ROC AUC: 0.921
ROC AUC: 0.916
ROC AUC: 0.922
ROC AUC: 0.916
Mean AUC: 0.9189
SD AUC: 0.0024
Plot Receiver Operator Characteristic Curve
fig, ax = plt.subplots(figsize=(6,6))
for i in range(5):
ax.plot(roc_fpr[i], roc_tpr[i], color='orange', linestyle='-')
ax.plot([0, 1], [0, 1], color='darkblue', linestyle='--')
ax.set_xlabel('False Positive Rate (1 - Specificity)')
ax.set_ylabel('True Positive Rate (Sensitivity)')
ax.text(0.55, 0.1, f'Mean AUC: {mean_auc:0.3f}', fontsize=12,
bbox=dict(facecolor='w', alpha=1.0))
ax.grid()
filename = path + '2d_roc.jpg'
plt.savefig(filename, dpi=300)
plt.show()
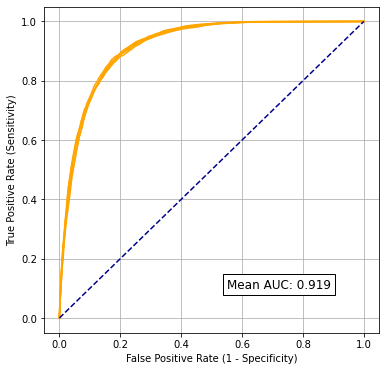
Plot Sensitivity-Specificity Curve alongside Receiver Operator Characteristic Curve
k_fold_sensitivity = []
k_fold_specificity = []
for i in range(5):
# Get classificiation probabilities for k-fold replicate
obs = observed[i]
proba = predicted_proba[i]
# Set up list for accuracy measures
sensitivity = []
specificity = []
# Loop through increments in probability of survival
thresholds = np.arange(0.0, 1.01, 0.01)
for cutoff in thresholds: # loop 0 --> 1 on steps of 0.1
# Get whether passengers survive using cutoff
predicted_class = proba >= cutoff
predicted_class = predicted_class * 1.0
# Call accuracy measures function
accuracy = calculate_accuracy(obs, predicted_class)
# Add accuracy scores to lists
sensitivity.append(accuracy['sensitivity'])
specificity.append(accuracy['specificity'])
# Add replicate to lists
k_fold_sensitivity.append(sensitivity)
k_fold_specificity.append(specificity)
fig = plt.figure(figsize=(10,5))
# Plot ROC
ax1 = fig.add_subplot(121)
for i in range(5):
ax1.plot(roc_fpr[i], roc_tpr[i], color='orange')
ax1.plot([0, 1], [0, 1], color='darkblue', linestyle='--')
ax1.set_xlabel('False Positive Rate')
ax1.set_ylabel('True Positive Rate')
ax1.set_title('Receiver Operator Characteristic Curve')
text = f'Mean AUC: {mean_auc:.3f}'
ax1.text(0.64,0.07, text,
bbox=dict(facecolor='white', edgecolor='black'))
plt.grid(True)
# Plot sensitivity-specificity
ax2 = fig.add_subplot(122)
for i in range(5):
ax2.plot(k_fold_sensitivity[i], k_fold_specificity[i])
ax2.set_xlabel('Sensitivity')
ax2.set_ylabel('Specificity')
ax2.set_title('Sensitivity-Specificity Curve')
plt.grid(True)
plt.tight_layout(pad=2)
plt.savefig('./output/nn_2d_roc_sens_spec.jpg', dpi=300)
plt.show()
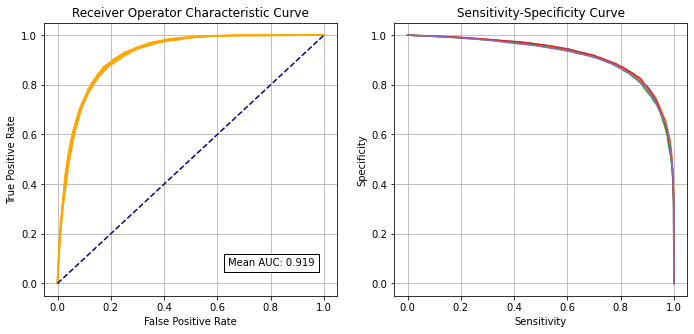
Identify cross-over of sensitivity and specificity#
sens = np.array(k_fold_sensitivity).mean(axis=0)
spec = np.array(k_fold_specificity).mean(axis=0)
df = pd.DataFrame()
df['sensitivity'] = sens
df['specificity'] = spec
df['spec greater sens'] = spec > sens
# find last index for senitivity being greater than specificity
mask = df['spec greater sens'] == False
last_id_sens_greater_spec = np.max(df[mask].index)
locs = [last_id_sens_greater_spec, last_id_sens_greater_spec + 1]
points = df.iloc[locs][['sensitivity', 'specificity']]
# Get intersetction with line of x=y
a1 = list(points.iloc[0].values)
a2 = list(points.iloc[1].values)
b1 = [0, 0]
b2 = [1, 1]
intersect = get_intersect(a1, a2, b1, b2)[0]
print(f'\nIntersect: {intersect:0.3f}')
Intersect: 0.842
Collate and save results#
hospital_results = []
kfold_result = []
threshold_results = []
observed_results = []
prob_results = []
predicted_results = []
for i in range(5):
hospital_results.extend(list(test_data[i]['StrokeTeam']))
kfold_result.extend(list(np.repeat(i, len(test_data[i]))))
threshold_results.extend(list(np.repeat(thresholds[i], len(test_data[i]))))
observed_results.extend(list(observed[i]))
prob_results.extend(list(predicted_proba[i]))
predicted_results.extend(list(predicted[i]))
model_results = pd.DataFrame()
model_results['hospital'] = hospital_results
model_results['observed'] = np.array(observed_results) * 1.0
model_results['prob'] = prob_results
model_results['predicted'] = predicted_results
model_results['k_fold'] = kfold_result
model_results['threshold'] = threshold_results
model_results['correct'] = model_results['observed'] == model_results['predicted']
# Save
filename = './predictions/nn_2d_k_fold.csv'
model_results.to_csv(filename, index=False)
Calibration and assessment of accuracy when model has high confidence#
# Collate results in Dataframe
reliability_collated = pd.DataFrame()
# Loop through k fold predictions
for i in range(5):
# Get observed class and predicted probability
obs = observed[i]
prob = predicted_proba[i]
# Bin data with numpy digitize (this will assign a bin to each case)
step = 0.10
bins = np.arange(step, 1+step, step)
digitized = np.digitize(prob, bins)
# Put single fold data in DataFrame
reliability = pd.DataFrame()
reliability['bin'] = digitized
reliability['probability'] = prob
reliability['observed'] = obs
classification = 1 * (prob > 0.5 )
reliability['correct'] = obs == classification
reliability['count'] = 1
# Summarise data by bin in new dataframe
reliability_summary = pd.DataFrame()
# Add bins and k-fold to summary
reliability_summary['bin'] = bins
reliability_summary['k-fold'] = i
# Calculate mean of predicted probability of thrombolysis in each bin
reliability_summary['confidence'] = \
reliability.groupby('bin').mean()['probability']
# Calculate the proportion of patients who receive thrombolysis
reliability_summary['fraction_positive'] = \
reliability.groupby('bin').mean()['observed']
# Calculate proportion correct in each bin
reliability_summary['fraction_correct'] = \
reliability.groupby('bin').mean()['correct']
# Calculate fraction of results in each bin
reliability_summary['fraction_results'] = \
reliability.groupby('bin').sum()['count'] / reliability.shape[0]
# Add k-fold results to DatafRame collation
reliability_collated = reliability_collated.append(reliability_summary)
# Get mean results
reliability_summary = reliability_collated.groupby('bin').mean()
reliability_summary.drop('k-fold', axis=1, inplace=True)
reliability_summary
confidence | fraction_positive | fraction_correct | fraction_results | |
---|---|---|---|---|
bin | ||||
0.1 | 0.022657 | 0.019716 | 0.980284 | 0.458776 |
0.2 | 0.145430 | 0.146271 | 0.853729 | 0.093008 |
0.3 | 0.247804 | 0.240951 | 0.759049 | 0.058351 |
0.4 | 0.349919 | 0.352014 | 0.647986 | 0.048545 |
0.5 | 0.449713 | 0.441913 | 0.558087 | 0.045318 |
0.6 | 0.550911 | 0.544515 | 0.544515 | 0.049220 |
0.7 | 0.652019 | 0.659190 | 0.659190 | 0.058441 |
0.8 | 0.753202 | 0.769957 | 0.769957 | 0.075960 |
0.9 | 0.847601 | 0.856525 | 0.856525 | 0.087768 |
1.0 | 0.923924 | 0.912707 | 0.912707 | 0.024615 |
fig = plt.figure(figsize=(10,5))
# Plot predicted prob vs fraction psotive
ax1 = fig.add_subplot(1,2,1)
# Loop through k-fold reliability results
for i in range(5):
mask = reliability_collated['k-fold'] == i
k_fold_result = reliability_collated[mask]
x = k_fold_result['confidence']
y = k_fold_result['fraction_positive']
ax1.plot(x,y, color='orange')
# Add 1:1 line
ax1.plot([0,1],[0,1], color='k', linestyle ='--')
# Refine plot
ax1.set_xlabel('Model probability')
ax1.set_ylabel('Fraction positive')
ax1.set_xlim(0, 1)
ax1.set_ylim(0, 1)
ax1.grid()
# Plot accuracy vs probability
ax2 = fig.add_subplot(1,2,2)
# Loop through k-fold reliability results
for i in range(5):
mask = reliability_collated['k-fold'] == i
k_fold_result = reliability_collated[mask]
x = k_fold_result['confidence']
y = k_fold_result['fraction_correct']
ax2.plot(x,y, color='orange')
# Refine plot
ax2.set_xlabel('Model probability')
ax2.set_ylabel('Fraction correct')
ax2.set_xlim(0, 1)
ax2.set_ylim(0, 1)
ax2.grid()
ax3 = ax2.twinx() # instantiate a second axes that shares the same x-axis
for i in range(5):
mask = reliability_collated['k-fold'] == i
k_fold_result = reliability_collated[mask]
x = k_fold_result['confidence']
y = k_fold_result['fraction_results']
ax3.plot(x,y, color='blue')
ax3.set_xlim(0, 1)
ax3.set_ylim(0, 0.5)
ax3.set_ylabel('Fraction of samples')
custom_lines = [Line2D([0], [0], color='orange', alpha=0.6, lw=2),
Line2D([0], [0], color='blue', alpha = 0.6,lw=2)]
plt.legend(custom_lines, ['Fraction correct', 'Fraction of samples'],
loc='upper center')
plt.tight_layout(pad=2)
plt.savefig('./output/nn_2d_reliability.jpg', dpi=300)
plt.show()
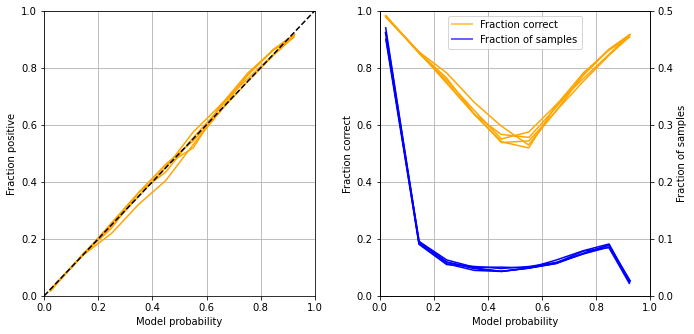
bins = [0.1, 0.2, 0.9, 1.0]
acc = reliability_summary.loc[bins].mean()['fraction_correct']
frac = reliability_summary.loc[bins].sum()['fraction_results']
print ('For samples with at least 80% confidence:')
print (f'Proportion of all samples: {frac:0.3f}')
print (f'Accuracy: {acc:0.3f}')
For samples with at least 80% confidence:
Proportion of all samples: 0.664
Accuracy: 0.901
Observations#
Overall accuracy = 85.2% (90.1% for those 66% samples with at least 80% confidence of model)
Using nominal threshold (50% probability), specificity (89%) is greater than sensitivity (75%)
The model can achieve 84.2% sensitivity and specificity simultaneously
ROC AUC = 0.919